1. 조건문
>>if문
if(조건식){
//조건식이 참일때 수행될 문장들을 적음
}
-블럭 {} 의 긑에는 ;를 붙이지 않음
- 블럭의 연산이 하나인 경우 {}를 사용하지 않아도 됨
but, 이후 수정과정이 까다로워질 수 있음
>예시
if(score>60)
System.out.println("합격입니다.") // if에 속한 문장
System.out.println("축하합니다.") // if에 속하지 않은 문장
>>숫자를 입력받아 분기하는 예제
import java.util.*;
public class Flowex2 {
public static void main(String[] args) {
Scanner scanner= new Scanner(System.in);
int input;
System.out.print("숫자를 하나 입력하세요 >");
String tmp= scanner.nextLine();
input = Integer.parseInt(tmp);
if (input==0)
{
System.out.println("입력하신 숫자는 0입니다.");
}
if(input !=0)
{
System.out.println("입력하신 숫자는 0이 아닙니다.");
}
System.out.printf("입력하신 숫자는 %d 입니다.%n", input);
}
}
>> else- if / else 문
>> 점수별 성적 분기 예제
import java.util.*;
public class Flowex4 {
public static void main(String[] args) {
int score;
char grade=' ';
Scanner scanner = new Scanner(System.in);
System.out.print("점수를 입력하세요 >");
score= scanner.nextInt();
if(score>=90)
{
grade='A';
}
else if(score>=80)
{
grade='B';
}
else if(score>=70)
{
grade='C';
}
else {
grade='D';
}
System.out.println("당신이 학점은 "+ grade +"입니다.");
}
}
>>Switch 문
- if문과 달리 int 타입의 정수와 문자열만 가능
- 계산결과와 일치하는 case로이동
- break문이나 switch문 끝을 만나면 switch문 전체를 빠져나감
-case문 : 리터럴, 상수, 문자열상수만 가능
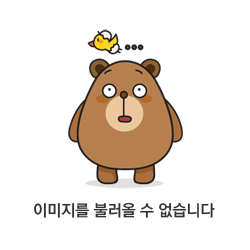
>switch문이 제약조건
1) switch 문 조건식 결과는 정수 또는 문자열
2) case문의 값은 정수 상수만 가능하며 중복되지 않아야 함
=> 변수나 실수 리터럴은 case문의 값으로 적합x
>>컴퓨터와 가위바위보 하는 예제
case를 통해 승리/패배 여부 판단
import java.util.*;
public class flowex7 {
public static void main(String[] args) {
int com, user;
Scanner scanner = new Scanner(System.in);
System.out.print("숫자를 입력해주세요 : 가위(1), 바위(2), 보(3) >");
user = scanner.nextInt();
com = (int)(Math.random()*3)+1;
System.out.printf("당신은 %d 입니다.%n", user);
System.out.printf("컴퓨터는 %d 입니다.%n", com);
switch(user-com)
{
case 2: case-1:
{
System.out.println("컴퓨터가 이겼습니다");
}
break;
case 1: case -2:
{
System.out.println("당신이 이겼습니다.");
}
break;
case 0:
{
System.out.println("비겼습니다.");
}
}
}
}
2. 반복문 - for, while, do-while
for
- 초기화, 조건식, 증감식 그리고 수행할 블록{} 으로 구성
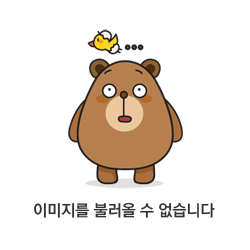
>>향상된 for문
: 배열이나 컬렉션을 차례대로 읽어오는데 사용
for( 타입 변수 명 : 배열/컬렉션)
{
//반복할 문장
}
ex)
int [] arr={1,2,3,4,5}
for( int tmp : arr) === python : for tmp in arr
{ (.......) }
ex)
public class flowex22 {
public static void main(String[] args) {
int[] arr={1,2,3,4,5};
for(int i=0; i<arr.length; i++)
{
System.out.printf("%d ", arr[i]);
}
System.out.println();
for( int i : arr)
{
System.out.printf("%d ", i);
}
System.out.println();
}
}
>>while문
- 조건식과 수행할 블럭 {} 또는 문장으로 구성
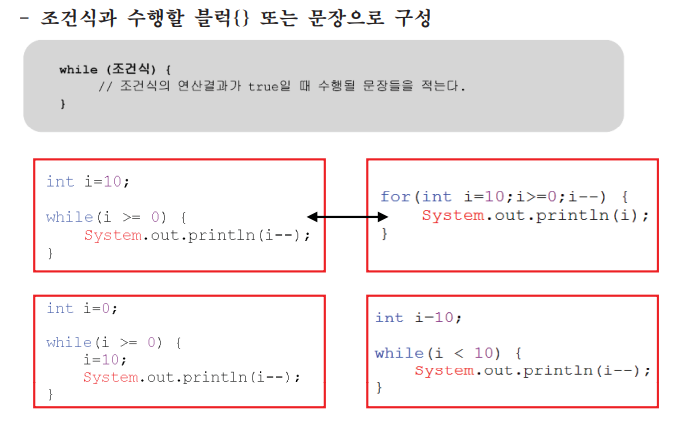
>>do-while문
: while문이 조건식과 블록 순서를 바꿔놓은 것
: 블록이 최소 한번 수행됨을 보장한다.
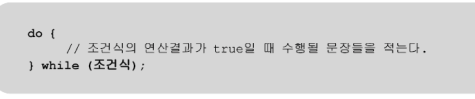
>> 예제 : 컴퓨터가 생각한 숫자를 맞추는 게임
import java.util.*;
public class flowex28 {
public static void main(String[] args) {
int tmp;
int com = (int)(Math.random()*100)+1;
Scanner scanner= new Scanner(System.in);
do {
System.out.printf("숫자를 입력하세요");
tmp = scanner.nextInt();
if (tmp> com)
{
System.out.println("더 작은 수임 ㅋ");
}
else if(tmp<com)
{
System.out.println("더 큰 수임 ㅋ");
}
}while(com!=tmp);
System.out.println("정답입니다.!");
}
}
>> 이름 붙은 반복문
: 반복문의 이름을 지정하여 break / continue가 가능함
=> c나 python 처럼 flag 지정을 하지 않아도 됨
public class flowex33 {
public static void main(String[] args) {
Loop1 : for(int i=2; i<10; i++)
{
for(int j=1; j<10; j++)
{
if (j==5)
{
break Loop1; //바깥 반복문을 벗어남
}
System.out.println(i+"*"+j+"="+i*j);
}
System.out.println();
}
}
}
>실행결과
j==5일 때 반복문 벗어남
2*1=2
2*2=4
2*3=6
2*4=8
'기술 서적 > 자바의 정석' 카테고리의 다른 글
[자바의 정석] ch8. 예외처리 (0) | 2024.01.06 |
---|---|
[자바의 정석] ch7. 객체지향 프로그래밍 II (0) | 2024.01.05 |
[자바의 정석] ch5. 배열 (0) | 2023.12.02 |
[자바의 정석] ch3. 연산자 (0) | 2023.10.08 |
[자바의 정석] ch2. 변수 (0) | 2023.10.07 |